mirror of
https://github.com/catchorg/Catch2.git
synced 2025-07-14 20:55:33 +02:00
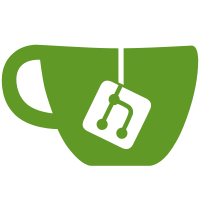
This prevents Clang from complaining about unused value in expressions containing explicit casts used in the THROW assertion macro family. Example: `REQUIRE_THROWS_AS(static_cast<bool>(object), std::bad_cast);` would trigger `-Wunused-value` warning. Now it does not. Credits to Arto Bendiken, who submitted a PR almost 3 years ago, but his branch has since died and I was unable to merge it.
144 lines
6.6 KiB
C++
144 lines
6.6 KiB
C++
/*
|
|
* Created by Phil on 18/10/2010.
|
|
* Copyright 2010 Two Blue Cubes Ltd. All rights reserved.
|
|
*
|
|
* Distributed under the Boost Software License, Version 1.0. (See accompanying
|
|
* file LICENSE_1_0.txt or copy at http://www.boost.org/LICENSE_1_0.txt)
|
|
*/
|
|
#ifndef TWOBLUECUBES_CATCH_CAPTURE_HPP_INCLUDED
|
|
#define TWOBLUECUBES_CATCH_CAPTURE_HPP_INCLUDED
|
|
|
|
#include "catch_result_builder.h"
|
|
#include "catch_message.h"
|
|
#include "catch_interfaces_capture.h"
|
|
#include "catch_debugger.h"
|
|
#include "catch_common.h"
|
|
#include "catch_tostring.h"
|
|
#include "catch_interfaces_runner.h"
|
|
#include "catch_compiler_capabilities.h"
|
|
|
|
|
|
///////////////////////////////////////////////////////////////////////////////
|
|
// In the event of a failure works out if the debugger needs to be invoked
|
|
// and/or an exception thrown and takes appropriate action.
|
|
// This needs to be done as a macro so the debugger will stop in the user
|
|
// source code rather than in Catch library code
|
|
#define INTERNAL_CATCH_REACT( resultBuilder ) \
|
|
if( resultBuilder.shouldDebugBreak() ) CATCH_BREAK_INTO_DEBUGGER(); \
|
|
resultBuilder.react();
|
|
|
|
|
|
///////////////////////////////////////////////////////////////////////////////
|
|
#define INTERNAL_CATCH_TEST( expr, resultDisposition, macroName ) \
|
|
do { \
|
|
Catch::ResultBuilder __catchResult( macroName, CATCH_INTERNAL_LINEINFO, #expr, resultDisposition ); \
|
|
try { \
|
|
CATCH_INTERNAL_SUPPRESS_PARENTHESES_WARNINGS \
|
|
( __catchResult <= expr ).endExpression(); \
|
|
} \
|
|
catch( ... ) { \
|
|
__catchResult.useActiveException( resultDisposition ); \
|
|
} \
|
|
INTERNAL_CATCH_REACT( __catchResult ) \
|
|
} while( Catch::isTrue( false && static_cast<bool>( !!(expr) ) ) ) // expr here is never evaluated at runtime but it forces the compiler to give it a look
|
|
// The double negation silences MSVC's C4800 warning, the static_cast forces short-circuit evaluation if the type has overloaded &&.
|
|
|
|
///////////////////////////////////////////////////////////////////////////////
|
|
#define INTERNAL_CATCH_IF( expr, resultDisposition, macroName ) \
|
|
INTERNAL_CATCH_TEST( expr, resultDisposition, macroName ); \
|
|
if( Catch::getResultCapture().getLastResult()->succeeded() )
|
|
|
|
///////////////////////////////////////////////////////////////////////////////
|
|
#define INTERNAL_CATCH_ELSE( expr, resultDisposition, macroName ) \
|
|
INTERNAL_CATCH_TEST( expr, resultDisposition, macroName ); \
|
|
if( !Catch::getResultCapture().getLastResult()->succeeded() )
|
|
|
|
///////////////////////////////////////////////////////////////////////////////
|
|
#define INTERNAL_CATCH_NO_THROW( expr, resultDisposition, macroName ) \
|
|
do { \
|
|
Catch::ResultBuilder __catchResult( macroName, CATCH_INTERNAL_LINEINFO, #expr, resultDisposition ); \
|
|
try { \
|
|
static_cast<void>(expr); \
|
|
__catchResult.captureResult( Catch::ResultWas::Ok ); \
|
|
} \
|
|
catch( ... ) { \
|
|
__catchResult.useActiveException( resultDisposition ); \
|
|
} \
|
|
INTERNAL_CATCH_REACT( __catchResult ) \
|
|
} while( Catch::alwaysFalse() )
|
|
|
|
///////////////////////////////////////////////////////////////////////////////
|
|
#define INTERNAL_CATCH_THROWS( expr, resultDisposition, matcher, macroName ) \
|
|
do { \
|
|
Catch::ResultBuilder __catchResult( macroName, CATCH_INTERNAL_LINEINFO, #expr, resultDisposition, #matcher ); \
|
|
if( __catchResult.allowThrows() ) \
|
|
try { \
|
|
static_cast<void>(expr); \
|
|
__catchResult.captureResult( Catch::ResultWas::DidntThrowException ); \
|
|
} \
|
|
catch( ... ) { \
|
|
__catchResult.captureExpectedException( matcher ); \
|
|
} \
|
|
else \
|
|
__catchResult.captureResult( Catch::ResultWas::Ok ); \
|
|
INTERNAL_CATCH_REACT( __catchResult ) \
|
|
} while( Catch::alwaysFalse() )
|
|
|
|
///////////////////////////////////////////////////////////////////////////////
|
|
#define INTERNAL_CATCH_THROWS_AS( expr, exceptionType, resultDisposition, macroName ) \
|
|
do { \
|
|
Catch::ResultBuilder __catchResult( macroName, CATCH_INTERNAL_LINEINFO, #expr, resultDisposition ); \
|
|
if( __catchResult.allowThrows() ) \
|
|
try { \
|
|
static_cast<void>(expr); \
|
|
__catchResult.captureResult( Catch::ResultWas::DidntThrowException ); \
|
|
} \
|
|
catch( exceptionType ) { \
|
|
__catchResult.captureResult( Catch::ResultWas::Ok ); \
|
|
} \
|
|
catch( ... ) { \
|
|
__catchResult.useActiveException( resultDisposition ); \
|
|
} \
|
|
else \
|
|
__catchResult.captureResult( Catch::ResultWas::Ok ); \
|
|
INTERNAL_CATCH_REACT( __catchResult ) \
|
|
} while( Catch::alwaysFalse() )
|
|
|
|
|
|
///////////////////////////////////////////////////////////////////////////////
|
|
#ifdef CATCH_CONFIG_VARIADIC_MACROS
|
|
#define INTERNAL_CATCH_MSG( messageType, resultDisposition, macroName, ... ) \
|
|
do { \
|
|
Catch::ResultBuilder __catchResult( macroName, CATCH_INTERNAL_LINEINFO, "", resultDisposition ); \
|
|
__catchResult << __VA_ARGS__ + ::Catch::StreamEndStop(); \
|
|
__catchResult.captureResult( messageType ); \
|
|
INTERNAL_CATCH_REACT( __catchResult ) \
|
|
} while( Catch::alwaysFalse() )
|
|
#else
|
|
#define INTERNAL_CATCH_MSG( messageType, resultDisposition, macroName, log ) \
|
|
do { \
|
|
Catch::ResultBuilder __catchResult( macroName, CATCH_INTERNAL_LINEINFO, "", resultDisposition ); \
|
|
__catchResult << log + ::Catch::StreamEndStop(); \
|
|
__catchResult.captureResult( messageType ); \
|
|
INTERNAL_CATCH_REACT( __catchResult ) \
|
|
} while( Catch::alwaysFalse() )
|
|
#endif
|
|
|
|
///////////////////////////////////////////////////////////////////////////////
|
|
#define INTERNAL_CATCH_INFO( log, macroName ) \
|
|
Catch::ScopedMessage INTERNAL_CATCH_UNIQUE_NAME( scopedMessage ) = Catch::MessageBuilder( macroName, CATCH_INTERNAL_LINEINFO, Catch::ResultWas::Info ) << log;
|
|
|
|
///////////////////////////////////////////////////////////////////////////////
|
|
#define INTERNAL_CHECK_THAT( arg, matcher, resultDisposition, macroName ) \
|
|
do { \
|
|
Catch::ResultBuilder __catchResult( macroName, CATCH_INTERNAL_LINEINFO, #arg ", " #matcher, resultDisposition ); \
|
|
try { \
|
|
__catchResult.captureMatch( arg, matcher, #matcher ); \
|
|
} catch( ... ) { \
|
|
__catchResult.useActiveException( resultDisposition | Catch::ResultDisposition::ContinueOnFailure ); \
|
|
} \
|
|
INTERNAL_CATCH_REACT( __catchResult ) \
|
|
} while( Catch::alwaysFalse() )
|
|
|
|
#endif // TWOBLUECUBES_CATCH_CAPTURE_HPP_INCLUDED
|