mirror of
https://github.com/catchorg/Catch2.git
synced 2024-10-25 18:14:42 +02:00
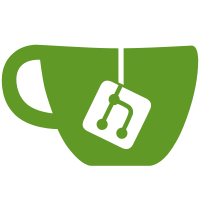
* Empty strings are now direct constructed as `std::string()`, not as empty string literals. * `startsWith` and `endsWith` no longer construct new a string. This should be an improvement for libstdc++ when using older standards, as it doesn't use SSO but COW and thus even short strings are expensive to first create. * Various places now use char literal instead of string literals containing single char. ** `startsWith` and `endsWith` now also have overload that takes single char. Generally the performance improvements under VS2015 are small, as going from short string to char is mostly meaningless because of SSO (Catch doesn't push string handling that hard) and previous commit removed most string handling if tests pass, which is the expect case.
72 lines
2.4 KiB
C++
72 lines
2.4 KiB
C++
/*
|
|
* Created by Phil on 13/7/2015.
|
|
* Copyright 2015 Two Blue Cubes Ltd. All rights reserved.
|
|
*
|
|
* Distributed under the Boost Software License, Version 1.0. (See accompanying
|
|
* file LICENSE_1_0.txt or copy at http://www.boost.org/LICENSE_1_0.txt)
|
|
*/
|
|
#ifndef TWOBLUECUBES_CATCH_WILDCARD_PATTERN_HPP_INCLUDED
|
|
#define TWOBLUECUBES_CATCH_WILDCARD_PATTERN_HPP_INCLUDED
|
|
|
|
#include "catch_common.h"
|
|
|
|
namespace Catch
|
|
{
|
|
class WildcardPattern {
|
|
enum WildcardPosition {
|
|
NoWildcard = 0,
|
|
WildcardAtStart = 1,
|
|
WildcardAtEnd = 2,
|
|
WildcardAtBothEnds = WildcardAtStart | WildcardAtEnd
|
|
};
|
|
|
|
public:
|
|
|
|
WildcardPattern( std::string const& pattern, CaseSensitive::Choice caseSensitivity )
|
|
: m_caseSensitivity( caseSensitivity ),
|
|
m_wildcard( NoWildcard ),
|
|
m_pattern( adjustCase( pattern ) )
|
|
{
|
|
if( startsWith( m_pattern, '*' ) ) {
|
|
m_pattern = m_pattern.substr( 1 );
|
|
m_wildcard = WildcardAtStart;
|
|
}
|
|
if( endsWith( m_pattern, '*' ) ) {
|
|
m_pattern = m_pattern.substr( 0, m_pattern.size()-1 );
|
|
m_wildcard = static_cast<WildcardPosition>( m_wildcard | WildcardAtEnd );
|
|
}
|
|
}
|
|
virtual ~WildcardPattern();
|
|
virtual bool matches( std::string const& str ) const {
|
|
switch( m_wildcard ) {
|
|
case NoWildcard:
|
|
return m_pattern == adjustCase( str );
|
|
case WildcardAtStart:
|
|
return endsWith( adjustCase( str ), m_pattern );
|
|
case WildcardAtEnd:
|
|
return startsWith( adjustCase( str ), m_pattern );
|
|
case WildcardAtBothEnds:
|
|
return contains( adjustCase( str ), m_pattern );
|
|
}
|
|
|
|
#ifdef __clang__
|
|
#pragma clang diagnostic push
|
|
#pragma clang diagnostic ignored "-Wunreachable-code"
|
|
#endif
|
|
throw std::logic_error( "Unknown enum" );
|
|
#ifdef __clang__
|
|
#pragma clang diagnostic pop
|
|
#endif
|
|
}
|
|
private:
|
|
std::string adjustCase( std::string const& str ) const {
|
|
return m_caseSensitivity == CaseSensitive::No ? toLower( str ) : str;
|
|
}
|
|
CaseSensitive::Choice m_caseSensitivity;
|
|
WildcardPosition m_wildcard;
|
|
std::string m_pattern;
|
|
};
|
|
}
|
|
|
|
#endif // TWOBLUECUBES_CATCH_WILDCARD_PATTERN_HPP_INCLUDED
|