mirror of
https://github.com/catchorg/Catch2.git
synced 2025-07-02 07:35:32 +02:00
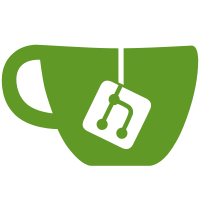
By using char const * instead of std::string we avoid significant copying per assertion. In a simple loop with 10000000 CHECKS on my system, this reduces the run time from 9.8s to 5.8s. This change is at the expense of no longer capturing the second arg, which no currently existing test notices.
100 lines
3.1 KiB
C++
100 lines
3.1 KiB
C++
/*
|
|
* Created by Phil on 8/8/12
|
|
* Copyright 2012 Two Blue Cubes Ltd. All rights reserved.
|
|
*
|
|
* Distributed under the Boost Software License, Version 1.0. (See accompanying
|
|
* file LICENSE_1_0.txt or copy at http://www.boost.org/LICENSE_1_0.txt)
|
|
*/
|
|
#ifndef TWOBLUECUBES_CATCH_ASSERTIONRESULT_HPP_INCLUDED
|
|
#define TWOBLUECUBES_CATCH_ASSERTIONRESULT_HPP_INCLUDED
|
|
|
|
#include "catch_assertionresult.h"
|
|
|
|
namespace Catch {
|
|
|
|
|
|
AssertionInfo::AssertionInfo( char const * _macroName,
|
|
SourceLineInfo const& _lineInfo,
|
|
char const * _capturedExpression,
|
|
ResultDisposition::Flags _resultDisposition )
|
|
: macroName( _macroName ),
|
|
lineInfo( _lineInfo ),
|
|
capturedExpression( _capturedExpression ),
|
|
resultDisposition( _resultDisposition )
|
|
{}
|
|
|
|
AssertionResult::AssertionResult() {}
|
|
|
|
AssertionResult::AssertionResult( AssertionInfo const& info, AssertionResultData const& data )
|
|
: m_info( info ),
|
|
m_resultData( data )
|
|
{}
|
|
|
|
AssertionResult::~AssertionResult() {}
|
|
|
|
// Result was a success
|
|
bool AssertionResult::succeeded() const {
|
|
return Catch::isOk( m_resultData.resultType );
|
|
}
|
|
|
|
// Result was a success, or failure is suppressed
|
|
bool AssertionResult::isOk() const {
|
|
return Catch::isOk( m_resultData.resultType ) || shouldSuppressFailure( m_info.resultDisposition );
|
|
}
|
|
|
|
ResultWas::OfType AssertionResult::getResultType() const {
|
|
return m_resultData.resultType;
|
|
}
|
|
|
|
bool AssertionResult::hasExpression() const {
|
|
return m_info.capturedExpression[0] != 0;
|
|
}
|
|
|
|
bool AssertionResult::hasMessage() const {
|
|
return !m_resultData.message.empty();
|
|
}
|
|
|
|
std::string AssertionResult::getExpression() const {
|
|
if( isFalseTest( m_info.resultDisposition ) )
|
|
return '!' + m_info.capturedExpression;
|
|
else
|
|
return m_info.capturedExpression;
|
|
}
|
|
std::string AssertionResult::getExpressionInMacro() const {
|
|
if( m_info.macroName[0] == 0 )
|
|
return m_info.capturedExpression;
|
|
else
|
|
return std::string(m_info.macroName) + "( " + m_info.capturedExpression + " )";
|
|
}
|
|
|
|
bool AssertionResult::hasExpandedExpression() const {
|
|
return hasExpression() && getExpandedExpression() != getExpression();
|
|
}
|
|
|
|
std::string AssertionResult::getExpandedExpression() const {
|
|
return m_resultData.reconstructExpression();
|
|
}
|
|
|
|
std::string AssertionResult::getMessage() const {
|
|
return m_resultData.message;
|
|
}
|
|
SourceLineInfo AssertionResult::getSourceInfo() const {
|
|
return m_info.lineInfo;
|
|
}
|
|
|
|
std::string AssertionResult::getTestMacroName() const {
|
|
return m_info.macroName;
|
|
}
|
|
|
|
void AssertionResult::discardDecomposedExpression() const {
|
|
m_resultData.decomposedExpression = CATCH_NULL;
|
|
}
|
|
|
|
void AssertionResult::expandDecomposedExpression() const {
|
|
m_resultData.reconstructExpression();
|
|
}
|
|
|
|
} // end namespace Catch
|
|
|
|
#endif // TWOBLUECUBES_CATCH_ASSERTIONRESULT_HPP_INCLUDED
|