mirror of
https://github.com/catchorg/Catch2.git
synced 2024-11-09 15:49:53 +01:00
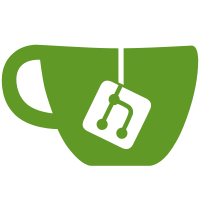
A test runner already has a --durations option to print durations. However, this isn't entirely satisfactory. When there are many tests, this produces output spam which makes it hard to find the test failure output. Nevertheless, it is helpful to be informed of tests which are unusually slow. Therefore, introduce a new option --min-duration that causes all durations above a certain threshold to be printed. This allows slow tests to be visible without mentioning every test.
81 lines
2.4 KiB
C++
81 lines
2.4 KiB
C++
/*
|
|
* Created by Phil on 27/11/2013.
|
|
* Copyright 2013 Two Blue Cubes Ltd. All rights reserved.
|
|
*
|
|
* Distributed under the Boost Software License, Version 1.0. (See accompanying
|
|
* file LICENSE_1_0.txt or copy at http://www.boost.org/LICENSE_1_0.txt)
|
|
*/
|
|
|
|
#include "../internal/catch_interfaces_reporter.h"
|
|
#include "../internal/catch_errno_guard.h"
|
|
#include "catch_reporter_bases.hpp"
|
|
|
|
#include <cstring>
|
|
#include <cfloat>
|
|
#include <cstdio>
|
|
#include <cassert>
|
|
#include <memory>
|
|
|
|
namespace Catch {
|
|
void prepareExpandedExpression(AssertionResult& result) {
|
|
result.getExpandedExpression();
|
|
}
|
|
|
|
// Because formatting using c++ streams is stateful, drop down to C is required
|
|
// Alternatively we could use stringstream, but its performance is... not good.
|
|
std::string getFormattedDuration( double duration ) {
|
|
// Max exponent + 1 is required to represent the whole part
|
|
// + 1 for decimal point
|
|
// + 3 for the 3 decimal places
|
|
// + 1 for null terminator
|
|
const std::size_t maxDoubleSize = DBL_MAX_10_EXP + 1 + 1 + 3 + 1;
|
|
char buffer[maxDoubleSize];
|
|
|
|
// Save previous errno, to prevent sprintf from overwriting it
|
|
ErrnoGuard guard;
|
|
#ifdef _MSC_VER
|
|
sprintf_s(buffer, "%.3f", duration);
|
|
#else
|
|
std::sprintf(buffer, "%.3f", duration);
|
|
#endif
|
|
return std::string(buffer);
|
|
}
|
|
|
|
bool shouldShowDuration( IConfig const& config, double duration ) {
|
|
if( config.showDurations() == ShowDurations::Always )
|
|
return true;
|
|
double min = config.minDuration();
|
|
return min >= 0 && duration >= min;
|
|
}
|
|
|
|
std::string serializeFilters( std::vector<std::string> const& container ) {
|
|
ReusableStringStream oss;
|
|
bool first = true;
|
|
for (auto&& filter : container)
|
|
{
|
|
if (!first)
|
|
oss << ' ';
|
|
else
|
|
first = false;
|
|
|
|
oss << filter;
|
|
}
|
|
return oss.str();
|
|
}
|
|
|
|
TestEventListenerBase::TestEventListenerBase(ReporterConfig const & _config)
|
|
:StreamingReporterBase(_config) {}
|
|
|
|
std::set<Verbosity> TestEventListenerBase::getSupportedVerbosities() {
|
|
return { Verbosity::Quiet, Verbosity::Normal, Verbosity::High };
|
|
}
|
|
|
|
void TestEventListenerBase::assertionStarting(AssertionInfo const &) {}
|
|
|
|
bool TestEventListenerBase::assertionEnded(AssertionStats const &) {
|
|
return false;
|
|
}
|
|
|
|
|
|
} // end namespace Catch
|