mirror of
https://github.com/catchorg/Catch2.git
synced 2024-10-26 02:24:42 +02:00
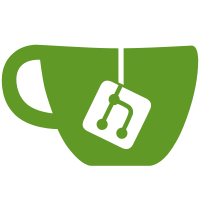
* Empty strings are now direct constructed as `std::string()`, not as empty string literals. * `startsWith` and `endsWith` no longer construct new a string. This should be an improvement for libstdc++ when using older standards, as it doesn't use SSO but COW and thus even short strings are expensive to first create. * Various places now use char literal instead of string literals containing single char. ** `startsWith` and `endsWith` now also have overload that takes single char. Generally the performance improvements under VS2015 are small, as going from short string to char is mostly meaningless because of SSO (Catch doesn't push string handling that hard) and previous commit removed most string handling if tests pass, which is the expect case.
112 lines
3.7 KiB
C++
112 lines
3.7 KiB
C++
/*
|
|
* Created by Phil on 28/5/2014.
|
|
* Copyright 2014 Two Blue Cubes Ltd. All rights reserved.
|
|
*
|
|
* Distributed under the Boost Software License, Version 1.0. (See accompanying
|
|
* file LICENSE_1_0.txt or copy at http://www.boost.org/LICENSE_1_0.txt)
|
|
*/
|
|
#ifndef TWOBLUECUBES_CATCH_RESULT_BUILDER_H_INCLUDED
|
|
#define TWOBLUECUBES_CATCH_RESULT_BUILDER_H_INCLUDED
|
|
|
|
#include "catch_result_type.h"
|
|
#include "catch_assertionresult.h"
|
|
#include "catch_common.h"
|
|
#include "catch_matchers.hpp"
|
|
|
|
namespace Catch {
|
|
|
|
struct TestFailureException{};
|
|
|
|
template<typename T> class ExpressionLhs;
|
|
|
|
struct CopyableStream {
|
|
CopyableStream() {}
|
|
CopyableStream( CopyableStream const& other ) {
|
|
oss << other.oss.str();
|
|
}
|
|
CopyableStream& operator=( CopyableStream const& other ) {
|
|
oss.str(std::string());
|
|
oss << other.oss.str();
|
|
return *this;
|
|
}
|
|
std::ostringstream oss;
|
|
};
|
|
|
|
class ResultBuilder : public DecomposedExpression {
|
|
public:
|
|
ResultBuilder( char const* macroName,
|
|
SourceLineInfo const& lineInfo,
|
|
char const* capturedExpression,
|
|
ResultDisposition::Flags resultDisposition,
|
|
char const* secondArg = "" );
|
|
|
|
template<typename T>
|
|
ExpressionLhs<T const&> operator <= ( T const& operand );
|
|
ExpressionLhs<bool> operator <= ( bool value );
|
|
|
|
template<typename T>
|
|
ResultBuilder& operator << ( T const& value ) {
|
|
m_stream.oss << value;
|
|
return *this;
|
|
}
|
|
|
|
ResultBuilder& setResultType( ResultWas::OfType result );
|
|
ResultBuilder& setResultType( bool result );
|
|
|
|
void endExpression( DecomposedExpression const& expr );
|
|
|
|
virtual void reconstructExpression( std::string& dest ) const CATCH_OVERRIDE;
|
|
|
|
AssertionResult build() const;
|
|
AssertionResult build( DecomposedExpression const& expr ) const;
|
|
|
|
void useActiveException( ResultDisposition::Flags resultDisposition = ResultDisposition::Normal );
|
|
void captureResult( ResultWas::OfType resultType );
|
|
void captureExpression();
|
|
void captureExpectedException( std::string const& expectedMessage );
|
|
void captureExpectedException( Matchers::Impl::Matcher<std::string> const& matcher );
|
|
void handleResult( AssertionResult const& result );
|
|
void react();
|
|
bool shouldDebugBreak() const;
|
|
bool allowThrows() const;
|
|
|
|
template<typename ArgT, typename MatcherT>
|
|
void captureMatch( ArgT const& arg, MatcherT const& matcher, char const* matcherString );
|
|
|
|
private:
|
|
AssertionInfo m_assertionInfo;
|
|
AssertionResultData m_data;
|
|
CopyableStream m_stream;
|
|
|
|
bool m_shouldDebugBreak;
|
|
bool m_shouldThrow;
|
|
};
|
|
|
|
} // namespace Catch
|
|
|
|
// Include after due to circular dependency:
|
|
#include "catch_expression_lhs.hpp"
|
|
|
|
namespace Catch {
|
|
|
|
template<typename T>
|
|
inline ExpressionLhs<T const&> ResultBuilder::operator <= ( T const& operand ) {
|
|
return ExpressionLhs<T const&>( *this, operand );
|
|
}
|
|
|
|
inline ExpressionLhs<bool> ResultBuilder::operator <= ( bool value ) {
|
|
return ExpressionLhs<bool>( *this, value );
|
|
}
|
|
|
|
template<typename ArgT, typename MatcherT>
|
|
inline void ResultBuilder::captureMatch( ArgT const& arg, MatcherT const& matcher,
|
|
char const* matcherString ) {
|
|
MatchExpression<ArgT const&, MatcherT const&> expr( arg, matcher, matcherString );
|
|
setResultType( matcher.match( arg ) );
|
|
endExpression( expr );
|
|
}
|
|
|
|
} // namespace Catch
|
|
|
|
#endif // TWOBLUECUBES_CATCH_RESULT_BUILDER_H_INCLUDED
|