mirror of
https://github.com/catchorg/Catch2.git
synced 2024-11-04 05:09:53 +01:00
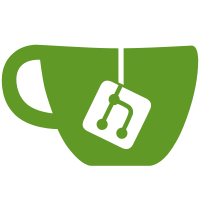
This also required some refactoring of how the pattern matching works. This means that the concepts of include and exclude patterns are no longer unified, with exclusion patterns working as just negation of an inclusion patterns (which led to including hidden tags by default, as they did not match the exclusion), but rather both include and exclude patterns are handled separately. The new logic is that given a filter and a test case, the test case must match _all_ include patterns and _no_ exclude patterns to be included by the filter. Furthermore, if the test case is hidden, then the filter must have at least one include pattern for the test case to be used. Closes #1184
88 lines
2.5 KiB
C++
88 lines
2.5 KiB
C++
/*
|
|
* Created by Phil on 14/8/2012.
|
|
* Copyright 2010 Two Blue Cubes Ltd. All rights reserved.
|
|
*
|
|
* Distributed under the Boost Software License, Version 1.0. (See accompanying
|
|
* file LICENSE_1_0.txt or copy at http://www.boost.org/LICENSE_1_0.txt)
|
|
*/
|
|
#ifndef TWOBLUECUBES_CATCH_TEST_SPEC_HPP_INCLUDED
|
|
#define TWOBLUECUBES_CATCH_TEST_SPEC_HPP_INCLUDED
|
|
|
|
#ifdef __clang__
|
|
#pragma clang diagnostic push
|
|
#pragma clang diagnostic ignored "-Wpadded"
|
|
#endif
|
|
|
|
#include "catch_wildcard_pattern.h"
|
|
#include "catch_test_case_info.h"
|
|
|
|
#include <string>
|
|
#include <vector>
|
|
#include <memory>
|
|
|
|
namespace Catch {
|
|
|
|
struct IConfig;
|
|
|
|
class TestSpec {
|
|
class Pattern {
|
|
public:
|
|
explicit Pattern( std::string const& name );
|
|
virtual ~Pattern();
|
|
virtual bool matches( TestCaseInfo const& testCase ) const = 0;
|
|
std::string const& name() const;
|
|
private:
|
|
std::string const m_name;
|
|
};
|
|
using PatternPtr = std::shared_ptr<Pattern>;
|
|
|
|
class NamePattern : public Pattern {
|
|
public:
|
|
explicit NamePattern( std::string const& name, std::string const& filterString );
|
|
bool matches( TestCaseInfo const& testCase ) const override;
|
|
private:
|
|
WildcardPattern m_wildcardPattern;
|
|
};
|
|
|
|
class TagPattern : public Pattern {
|
|
public:
|
|
explicit TagPattern( std::string const& tag, std::string const& filterString );
|
|
bool matches( TestCaseInfo const& testCase ) const override;
|
|
private:
|
|
std::string m_tag;
|
|
};
|
|
|
|
struct Filter {
|
|
std::vector<PatternPtr> m_required;
|
|
std::vector<PatternPtr> m_forbidden;
|
|
|
|
bool matches( TestCaseInfo const& testCase ) const;
|
|
std::string name() const;
|
|
};
|
|
|
|
public:
|
|
struct FilterMatch {
|
|
std::string name;
|
|
std::vector<TestCase const*> tests;
|
|
};
|
|
using Matches = std::vector<FilterMatch>;
|
|
using vectorStrings = std::vector<std::string>;
|
|
|
|
bool hasFilters() const;
|
|
bool matches( TestCaseInfo const& testCase ) const;
|
|
Matches matchesByFilter( std::vector<TestCase> const& testCases, IConfig const& config ) const;
|
|
const vectorStrings & getInvalidArgs() const;
|
|
|
|
private:
|
|
std::vector<Filter> m_filters;
|
|
std::vector<std::string> m_invalidArgs;
|
|
friend class TestSpecParser;
|
|
};
|
|
}
|
|
|
|
#ifdef __clang__
|
|
#pragma clang diagnostic pop
|
|
#endif
|
|
|
|
#endif // TWOBLUECUBES_CATCH_TEST_SPEC_HPP_INCLUDED
|