mirror of
https://github.com/catchorg/Catch2.git
synced 2024-11-04 05:09:53 +01:00
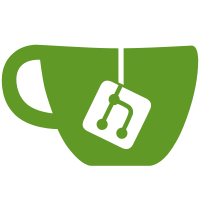
Previously, some errors in Catch configuration would cause exceptions to be thrown before main was even entered. This leads to call to `std::terminate`, which is not a particularly nice way of ending the binary. Now these exceptions are registered with a global collector and used once Catch enters main. They can also be optionally ignored, if user supplies his own main and opts not to check them (or ignored them intentionally). Closes #921
134 lines
4.5 KiB
C++
134 lines
4.5 KiB
C++
/*
|
|
* Created by Phil on 29/10/2010.
|
|
* Copyright 2010 Two Blue Cubes Ltd. All rights reserved.
|
|
*
|
|
* Distributed under the Boost Software License, Version 1.0. (See accompanying
|
|
* file LICENSE_1_0.txt or copy at http://www.boost.org/LICENSE_1_0.txt)
|
|
*/
|
|
#ifndef TWOBLUECUBES_CATCH_COMMON_H_INCLUDED
|
|
#define TWOBLUECUBES_CATCH_COMMON_H_INCLUDED
|
|
|
|
#include "catch_compiler_capabilities.h"
|
|
|
|
#define INTERNAL_CATCH_UNIQUE_NAME_LINE2( name, line ) name##line
|
|
#define INTERNAL_CATCH_UNIQUE_NAME_LINE( name, line ) INTERNAL_CATCH_UNIQUE_NAME_LINE2( name, line )
|
|
#ifdef CATCH_CONFIG_COUNTER
|
|
# define INTERNAL_CATCH_UNIQUE_NAME( name ) INTERNAL_CATCH_UNIQUE_NAME_LINE( name, __COUNTER__ )
|
|
#else
|
|
# define INTERNAL_CATCH_UNIQUE_NAME( name ) INTERNAL_CATCH_UNIQUE_NAME_LINE( name, __LINE__ )
|
|
#endif
|
|
|
|
#define INTERNAL_CATCH_STRINGIFY2( expr ) #expr
|
|
#define INTERNAL_CATCH_STRINGIFY( expr ) INTERNAL_CATCH_STRINGIFY2( expr )
|
|
|
|
#include <sstream>
|
|
#include <algorithm>
|
|
#include <exception>
|
|
|
|
namespace Catch {
|
|
|
|
struct IConfig;
|
|
|
|
struct CaseSensitive { enum Choice {
|
|
Yes,
|
|
No
|
|
}; };
|
|
|
|
class NonCopyable {
|
|
NonCopyable( NonCopyable const& ) = delete;
|
|
NonCopyable( NonCopyable && ) = delete;
|
|
NonCopyable& operator = ( NonCopyable const& ) = delete;
|
|
NonCopyable& operator = ( NonCopyable && ) = delete;
|
|
|
|
protected:
|
|
NonCopyable() {}
|
|
virtual ~NonCopyable();
|
|
};
|
|
|
|
template<typename ContainerT>
|
|
inline void deleteAll( ContainerT& container ) {
|
|
for( auto p : container )
|
|
delete p;
|
|
}
|
|
template<typename AssociativeContainerT>
|
|
inline void deleteAllValues( AssociativeContainerT& container ) {
|
|
for( auto const& kvp : container )
|
|
delete kvp.second;
|
|
}
|
|
|
|
bool startsWith( std::string const& s, std::string const& prefix );
|
|
bool startsWith( std::string const& s, char prefix );
|
|
bool endsWith( std::string const& s, std::string const& suffix );
|
|
bool endsWith( std::string const& s, char suffix );
|
|
bool contains( std::string const& s, std::string const& infix );
|
|
void toLowerInPlace( std::string& s );
|
|
std::string toLower( std::string const& s );
|
|
std::string trim( std::string const& str );
|
|
bool replaceInPlace( std::string& str, std::string const& replaceThis, std::string const& withThis );
|
|
|
|
struct pluralise {
|
|
pluralise( std::size_t count, std::string const& label );
|
|
|
|
friend std::ostream& operator << ( std::ostream& os, pluralise const& pluraliser );
|
|
|
|
std::size_t m_count;
|
|
std::string m_label;
|
|
};
|
|
|
|
struct SourceLineInfo {
|
|
|
|
SourceLineInfo();
|
|
SourceLineInfo( char const* _file, std::size_t _line );
|
|
|
|
SourceLineInfo(SourceLineInfo const& other) = default;
|
|
SourceLineInfo( SourceLineInfo && ) = default;
|
|
SourceLineInfo& operator = ( SourceLineInfo const& ) = default;
|
|
SourceLineInfo& operator = ( SourceLineInfo && ) = default;
|
|
|
|
bool empty() const;
|
|
bool operator == ( SourceLineInfo const& other ) const;
|
|
bool operator < ( SourceLineInfo const& other ) const;
|
|
|
|
char const* file;
|
|
std::size_t line;
|
|
};
|
|
|
|
std::ostream& operator << ( std::ostream& os, SourceLineInfo const& info );
|
|
|
|
// This is just here to avoid compiler warnings with macro constants and boolean literals
|
|
inline bool isTrue( bool value ){ return value; }
|
|
inline bool alwaysTrue() { return true; }
|
|
inline bool alwaysFalse() { return false; }
|
|
|
|
void seedRng( IConfig const& config );
|
|
unsigned int rngSeed();
|
|
|
|
// Use this in variadic streaming macros to allow
|
|
// >> +StreamEndStop
|
|
// as well as
|
|
// >> stuff +StreamEndStop
|
|
struct StreamEndStop {
|
|
std::string operator+() {
|
|
return std::string();
|
|
}
|
|
};
|
|
template<typename T>
|
|
T const& operator + ( T const& value, StreamEndStop ) {
|
|
return value;
|
|
}
|
|
}
|
|
|
|
#define CATCH_INTERNAL_LINEINFO \
|
|
::Catch::SourceLineInfo( __FILE__, static_cast<std::size_t>( __LINE__ ) )
|
|
#define CATCH_PREPARE_EXCEPTION( type, msg ) \
|
|
type( static_cast<std::ostringstream&&>( std::ostringstream() << msg ).str() )
|
|
#define CATCH_INTERNAL_ERROR( msg ) \
|
|
throw CATCH_PREPARE_EXCEPTION( std::logic_error, CATCH_INTERNAL_LINEINFO << ": Internal Catch error: " << msg);
|
|
#define CATCH_ERROR( msg ) \
|
|
throw CATCH_PREPARE_EXCEPTION( std::domain_error, msg )
|
|
#define CATCH_ENFORCE( condition, msg ) \
|
|
do{ if( !(condition) ) CATCH_ERROR( msg ); } while(false)
|
|
|
|
#endif // TWOBLUECUBES_CATCH_COMMON_H_INCLUDED
|
|
|