mirror of
https://github.com/catchorg/Catch2.git
synced 2024-10-25 18:14:42 +02:00
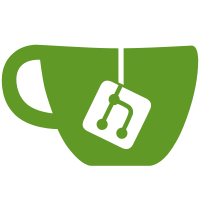
Some files had include guards that didn't match the file name, and others were missing the include guards entirely. Standardized this so that every include file has an include guard, and all the guards are of the form TWOBLUECUBES_<FILENAME>_<EXT>_INCLUDED
75 lines
2.1 KiB
C++
75 lines
2.1 KiB
C++
/*
|
|
* Created by Phil on 20/04/2011.
|
|
* Copyright 2011 Two Blue Cubes Ltd. All rights reserved.
|
|
*
|
|
* Distributed under the Boost Software License, Version 1.0. (See accompanying
|
|
* file LICENSE_1_0.txt or copy at http://www.boost.org/LICENSE_1_0.txt)
|
|
*/
|
|
#ifndef TWOBLUECUBES_CATCH_EXCEPTION_TRANSLATOR_REGISTRY_HPP_INCLUDED
|
|
#define TWOBLUECUBES_CATCH_EXCEPTION_TRANSLATOR_REGISTRY_HPP_INCLUDED
|
|
|
|
#include "catch_interfaces_exception.h"
|
|
|
|
#ifdef __OBJC__
|
|
#import "Foundation/Foundation.h"
|
|
#endif
|
|
|
|
namespace Catch {
|
|
|
|
class ExceptionTranslatorRegistry : public IExceptionTranslatorRegistry {
|
|
public:
|
|
~ExceptionTranslatorRegistry() {
|
|
deleteAll( m_translators );
|
|
}
|
|
|
|
virtual void registerTranslator( const IExceptionTranslator* translator ) {
|
|
m_translators.push_back( translator );
|
|
}
|
|
|
|
virtual std::string translateActiveException() const {
|
|
try {
|
|
#ifdef __OBJC__
|
|
// In Objective-C try objective-c exceptions first
|
|
@try {
|
|
throw;
|
|
}
|
|
@catch (NSException *exception) {
|
|
return toString( [exception description] );
|
|
}
|
|
#else
|
|
throw;
|
|
#endif
|
|
}
|
|
catch( std::exception& ex ) {
|
|
return ex.what();
|
|
}
|
|
catch( std::string& msg ) {
|
|
return msg;
|
|
}
|
|
catch( const char* msg ) {
|
|
return msg;
|
|
}
|
|
catch(...) {
|
|
return tryTranslators( m_translators.begin() );
|
|
}
|
|
}
|
|
|
|
std::string tryTranslators( std::vector<const IExceptionTranslator*>::const_iterator it ) const {
|
|
if( it == m_translators.end() )
|
|
return "Unknown exception";
|
|
|
|
try {
|
|
return (*it)->translate();
|
|
}
|
|
catch(...) {
|
|
return tryTranslators( it+1 );
|
|
}
|
|
}
|
|
|
|
private:
|
|
std::vector<const IExceptionTranslator*> m_translators;
|
|
};
|
|
}
|
|
|
|
#endif // TWOBLUECUBES_CATCH_EXCEPTION_TRANSLATOR_REGISTRY_HPP_INCLUDED
|