mirror of
https://github.com/catchorg/Catch2.git
synced 2024-10-25 18:14:42 +02:00
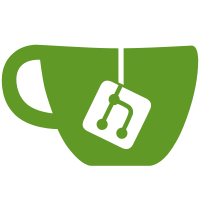
Some files had include guards that didn't match the file name, and others were missing the include guards entirely. Standardized this so that every include file has an include guard, and all the guards are of the form TWOBLUECUBES_<FILENAME>_<EXT>_INCLUDED
114 lines
3.1 KiB
Objective-C
114 lines
3.1 KiB
Objective-C
/*
|
|
* iTchRunnerReporter.h
|
|
* iTchRunner
|
|
*
|
|
* Created by Phil on 07/02/2011.
|
|
* Copyright 2011 Two Blue Cubes Ltd. All rights reserved.
|
|
*
|
|
*/
|
|
#ifndef TWOBLUECUBES_ITCHRUNNERREPORTER_H_INCLUDED
|
|
#define TWOBLUECUBES_ITCHRUNNERREPORTER_H_INCLUDED
|
|
|
|
#include "catch.hpp"
|
|
|
|
@protocol iTchRunnerDelegate
|
|
|
|
-(void) testWasRun: (const Catch::ResultInfo*) result;
|
|
|
|
@end
|
|
|
|
namespace Catch
|
|
{
|
|
class iTchRunnerReporter : public SharedImpl<IReporter>
|
|
{
|
|
public:
|
|
///////////////////////////////////////////////////////////////////////////
|
|
iTchRunnerReporter
|
|
(
|
|
id<iTchRunnerDelegate> delegate
|
|
)
|
|
: m_delegate( delegate )
|
|
{
|
|
}
|
|
|
|
///////////////////////////////////////////////////////////////////////////
|
|
virtual bool shouldRedirectStdout
|
|
()
|
|
const
|
|
{
|
|
return true;
|
|
}
|
|
|
|
///////////////////////////////////////////////////////////////////////////
|
|
static std::string getDescription
|
|
()
|
|
{
|
|
return "Captures results for iOS runner";
|
|
}
|
|
|
|
///////////////////////////////////////////////////////////////////////////
|
|
size_t getSucceeded
|
|
()
|
|
const
|
|
{
|
|
return m_totals.assertions.passed;
|
|
}
|
|
|
|
///////////////////////////////////////////////////////////////////////////
|
|
size_t getFailed
|
|
()
|
|
const
|
|
{
|
|
return m_totals.assertions.failed;
|
|
}
|
|
|
|
///////////////////////////////////////////////////////////////////////////
|
|
void reset()
|
|
{
|
|
m_totals = Totals();
|
|
}
|
|
|
|
private: // IReporter
|
|
|
|
///////////////////////////////////////////////////////////////////////////
|
|
virtual void StartTesting
|
|
()
|
|
{}
|
|
|
|
///////////////////////////////////////////////////////////////////////////
|
|
virtual void EndTesting
|
|
(
|
|
const Totals& totals
|
|
)
|
|
{
|
|
m_totals = totals;
|
|
}
|
|
|
|
///////////////////////////////////////////////////////////////////////////
|
|
virtual void Result
|
|
(
|
|
const ResultInfo& result
|
|
)
|
|
{
|
|
[m_delegate testWasRun: &result];
|
|
}
|
|
|
|
///////////////////////////////////////////////////////////////////////////
|
|
// Deliberately unimplemented:
|
|
virtual void StartGroup( const std::string& ){}
|
|
virtual void EndGroup( const std::string&, const Totals& ){}
|
|
virtual void StartTestCase( const TestCaseInfo& ){}
|
|
virtual void StartSection( const std::string& sectionName, const std::string& description ) {}
|
|
virtual void EndSection( const std::string&, const Counts& ){}
|
|
virtual void EndTestCase( const TestCaseInfo&, const Totals&, const std::string&, const std::string& ){}
|
|
virtual void Aborted() {}
|
|
|
|
private:
|
|
Totals m_totals;
|
|
|
|
id<iTchRunnerDelegate> m_delegate;
|
|
};
|
|
}
|
|
|
|
#endif // TWOBLUECUBES_ITCHRUNNERREPORTER_H_INCLUDED
|