mirror of
https://github.com/catchorg/Catch2.git
synced 2025-07-12 03:55:32 +02:00
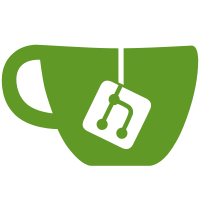
Previously, some errors in Catch configuration would cause exceptions to be thrown before main was even entered. This leads to call to `std::terminate`, which is not a particularly nice way of ending the binary. Now these exceptions are registered with a global collector and used once Catch enters main. They can also be optionally ignored, if user supplies his own main and opts not to check them (or ignored them intentionally). Closes #921
60 lines
2.1 KiB
C++
60 lines
2.1 KiB
C++
/*
|
|
* Created by Phil on 5/8/2012.
|
|
* Copyright 2012 Two Blue Cubes Ltd. All rights reserved.
|
|
*
|
|
* Distributed under the Boost Software License, Version 1.0. (See accompanying
|
|
* file LICENSE_1_0.txt or copy at http://www.boost.org/LICENSE_1_0.txt)
|
|
*/
|
|
#ifndef TWOBLUECUBES_CATCH_INTERFACES_REGISTRY_HUB_H_INCLUDED
|
|
#define TWOBLUECUBES_CATCH_INTERFACES_REGISTRY_HUB_H_INCLUDED
|
|
|
|
#include "catch_common.h"
|
|
|
|
#include <string>
|
|
#include <memory>
|
|
|
|
namespace Catch {
|
|
|
|
class TestCase;
|
|
struct ITestCaseRegistry;
|
|
struct IExceptionTranslatorRegistry;
|
|
struct IExceptionTranslator;
|
|
struct IReporterRegistry;
|
|
struct IReporterFactory;
|
|
struct ITagAliasRegistry;
|
|
class StartupExceptionRegistry;
|
|
|
|
using IReporterFactoryPtr = std::shared_ptr<IReporterFactory>;
|
|
|
|
struct IRegistryHub {
|
|
virtual ~IRegistryHub();
|
|
|
|
virtual IReporterRegistry const& getReporterRegistry() const = 0;
|
|
virtual ITestCaseRegistry const& getTestCaseRegistry() const = 0;
|
|
virtual ITagAliasRegistry const& getTagAliasRegistry() const = 0;
|
|
|
|
virtual IExceptionTranslatorRegistry& getExceptionTranslatorRegistry() = 0;
|
|
|
|
|
|
virtual StartupExceptionRegistry const& getStartupExceptionRegistry() const = 0;
|
|
};
|
|
|
|
struct IMutableRegistryHub {
|
|
virtual ~IMutableRegistryHub();
|
|
virtual void registerReporter( std::string const& name, IReporterFactoryPtr const& factory ) = 0;
|
|
virtual void registerListener( IReporterFactoryPtr const& factory ) = 0;
|
|
virtual void registerTest( TestCase const& testInfo ) = 0;
|
|
virtual void registerTranslator( const IExceptionTranslator* translator ) = 0;
|
|
virtual void registerTagAlias( std::string const& alias, std::string const& tag, SourceLineInfo const& lineInfo ) = 0;
|
|
virtual void registerStartupException( std::exception_ptr const& exception ) = 0;
|
|
};
|
|
|
|
IRegistryHub& getRegistryHub();
|
|
IMutableRegistryHub& getMutableRegistryHub();
|
|
void cleanUp();
|
|
std::string translateActiveException();
|
|
|
|
}
|
|
|
|
#endif // TWOBLUECUBES_CATCH_INTERFACES_REGISTRY_HUB_H_INCLUDED
|