mirror of
https://github.com/catchorg/Catch2.git
synced 2024-11-04 05:09:53 +01:00
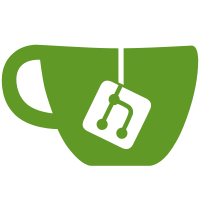
This also required some refactoring of how the pattern matching works. This means that the concepts of include and exclude patterns are no longer unified, with exclusion patterns working as just negation of an inclusion patterns (which led to including hidden tags by default, as they did not match the exclusion), but rather both include and exclude patterns are handled separately. The new logic is that given a filter and a test case, the test case must match _all_ include patterns and _no_ exclude patterns to be included by the filter. Furthermore, if the test case is hidden, then the filter must have at least one include pattern for the test case to be used. Closes #1184
96 lines
2.9 KiB
C++
96 lines
2.9 KiB
C++
/*
|
|
* Created by Phil on 15/5/2013.
|
|
* Copyright 2014 Two Blue Cubes Ltd. All rights reserved.
|
|
*
|
|
* Distributed under the Boost Software License, Version 1.0. (See accompanying
|
|
* file LICENSE_1_0.txt or copy at http://www.boost.org/LICENSE_1_0.txt)
|
|
*/
|
|
#ifndef TWOBLUECUBES_CATCH_TEST_SPEC_PARSER_HPP_INCLUDED
|
|
#define TWOBLUECUBES_CATCH_TEST_SPEC_PARSER_HPP_INCLUDED
|
|
|
|
#ifdef __clang__
|
|
#pragma clang diagnostic push
|
|
#pragma clang diagnostic ignored "-Wpadded"
|
|
#endif
|
|
|
|
#include "catch_test_spec.h"
|
|
#include "catch_string_manip.h"
|
|
#include "catch_interfaces_tag_alias_registry.h"
|
|
|
|
namespace Catch {
|
|
|
|
class TestSpecParser {
|
|
enum Mode{ None, Name, QuotedName, Tag, EscapedName };
|
|
Mode m_mode = None;
|
|
Mode lastMode = None;
|
|
bool m_exclusion = false;
|
|
std::size_t m_pos = 0;
|
|
std::size_t m_realPatternPos = 0;
|
|
std::string m_arg;
|
|
std::string m_substring;
|
|
std::string m_patternName;
|
|
std::vector<std::size_t> m_escapeChars;
|
|
TestSpec::Filter m_currentFilter;
|
|
TestSpec m_testSpec;
|
|
ITagAliasRegistry const* m_tagAliases = nullptr;
|
|
|
|
public:
|
|
TestSpecParser( ITagAliasRegistry const& tagAliases );
|
|
|
|
TestSpecParser& parse( std::string const& arg );
|
|
TestSpec testSpec();
|
|
|
|
private:
|
|
bool visitChar( char c );
|
|
void startNewMode( Mode mode );
|
|
bool processNoneChar( char c );
|
|
void processNameChar( char c );
|
|
bool processOtherChar( char c );
|
|
void endMode();
|
|
void escape();
|
|
bool isControlChar( char c ) const;
|
|
void saveLastMode();
|
|
void revertBackToLastMode();
|
|
void addFilter();
|
|
bool separate();
|
|
|
|
template<typename T>
|
|
void addPattern() {
|
|
std::string token = m_patternName;
|
|
for( std::size_t i = 0; i < m_escapeChars.size(); ++i )
|
|
token = token.substr( 0, m_escapeChars[i] - i ) + token.substr( m_escapeChars[i] -i +1 );
|
|
m_escapeChars.clear();
|
|
if( startsWith( token, "exclude:" ) ) {
|
|
m_exclusion = true;
|
|
token = token.substr( 8 );
|
|
}
|
|
if( !token.empty() ) {
|
|
TestSpec::PatternPtr pattern = std::make_shared<T>( token, m_substring );
|
|
if (m_exclusion) {
|
|
m_currentFilter.m_forbidden.push_back(pattern);
|
|
} else {
|
|
m_currentFilter.m_required.push_back(pattern);
|
|
}
|
|
}
|
|
m_substring.clear();
|
|
m_patternName.clear();
|
|
m_exclusion = false;
|
|
m_mode = None;
|
|
}
|
|
|
|
inline void addCharToPattern(char c) {
|
|
m_substring += c;
|
|
m_patternName += c;
|
|
m_realPatternPos++;
|
|
}
|
|
|
|
};
|
|
TestSpec parseTestSpec( std::string const& arg );
|
|
|
|
} // namespace Catch
|
|
|
|
#ifdef __clang__
|
|
#pragma clang diagnostic pop
|
|
#endif
|
|
|
|
#endif // TWOBLUECUBES_CATCH_TEST_SPEC_PARSER_HPP_INCLUDED
|