mirror of
https://github.com/catchorg/Catch2.git
synced 2024-11-04 05:09:53 +01:00
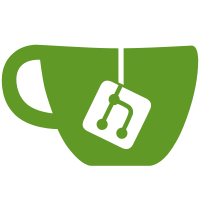
This PR ultimately does 3 things * Separately tracks matched tests per each filter part (that is, a set of filters separated by an OR (`,`)), which allows Catch2 to report each of the alternative filters that don't match any tests. * Fixes `-w NoTests` to return non-zero in the process * Adds tests for `-w NoTests`.
93 lines
2.6 KiB
C++
93 lines
2.6 KiB
C++
/*
|
|
* Created by Phil on 14/8/2012.
|
|
* Copyright 2010 Two Blue Cubes Ltd. All rights reserved.
|
|
*
|
|
* Distributed under the Boost Software License, Version 1.0. (See accompanying
|
|
* file LICENSE_1_0.txt or copy at http://www.boost.org/LICENSE_1_0.txt)
|
|
*/
|
|
#ifndef TWOBLUECUBES_CATCH_TEST_SPEC_HPP_INCLUDED
|
|
#define TWOBLUECUBES_CATCH_TEST_SPEC_HPP_INCLUDED
|
|
|
|
#ifdef __clang__
|
|
#pragma clang diagnostic push
|
|
#pragma clang diagnostic ignored "-Wpadded"
|
|
#endif
|
|
|
|
#include "catch_wildcard_pattern.h"
|
|
#include "catch_test_case_info.h"
|
|
|
|
#include <string>
|
|
#include <vector>
|
|
#include <memory>
|
|
|
|
namespace Catch {
|
|
|
|
struct IConfig;
|
|
|
|
class TestSpec {
|
|
class Pattern {
|
|
public:
|
|
explicit Pattern( std::string const& name );
|
|
virtual ~Pattern();
|
|
virtual bool matches( TestCaseInfo const& testCase ) const = 0;
|
|
std::string const& name() const;
|
|
private:
|
|
std::string const m_name;
|
|
};
|
|
using PatternPtr = std::shared_ptr<Pattern>;
|
|
|
|
class NamePattern : public Pattern {
|
|
public:
|
|
explicit NamePattern( std::string const& name, std::string const& filterString );
|
|
bool matches( TestCaseInfo const& testCase ) const override;
|
|
private:
|
|
WildcardPattern m_wildcardPattern;
|
|
};
|
|
|
|
class TagPattern : public Pattern {
|
|
public:
|
|
explicit TagPattern( std::string const& tag, std::string const& filterString );
|
|
bool matches( TestCaseInfo const& testCase ) const override;
|
|
private:
|
|
std::string m_tag;
|
|
};
|
|
|
|
class ExcludedPattern : public Pattern {
|
|
public:
|
|
explicit ExcludedPattern( PatternPtr const& underlyingPattern );
|
|
bool matches( TestCaseInfo const& testCase ) const override;
|
|
private:
|
|
PatternPtr m_underlyingPattern;
|
|
};
|
|
|
|
struct Filter {
|
|
std::vector<PatternPtr> m_patterns;
|
|
|
|
bool matches( TestCaseInfo const& testCase ) const;
|
|
std::string name() const;
|
|
};
|
|
|
|
public:
|
|
struct FilterMatch {
|
|
std::string name;
|
|
std::vector<TestCase const*> tests;
|
|
};
|
|
using Matches = std::vector<FilterMatch>;
|
|
|
|
bool hasFilters() const;
|
|
bool matches( TestCaseInfo const& testCase ) const;
|
|
Matches matchesByFilter( std::vector<TestCase> const& testCases, IConfig const& config ) const;
|
|
|
|
private:
|
|
std::vector<Filter> m_filters;
|
|
|
|
friend class TestSpecParser;
|
|
};
|
|
}
|
|
|
|
#ifdef __clang__
|
|
#pragma clang diagnostic pop
|
|
#endif
|
|
|
|
#endif // TWOBLUECUBES_CATCH_TEST_SPEC_HPP_INCLUDED
|